Spring Boot의 목적
Spring Boot는 다양한 기능을 통해, 애플리케이션을 프로덕션 환경에 빠르게 빌드하는 것을 돕습니다.
1. 빠르게 빌드
- Spring Initializr : 프로젝트를 빠르게 생성
- Spring Boot Starter Projects : 프로젝트의 의존성이 사전 정의 되어 있어서 필요에 따라 사용 가능
- REST API 빌드할 때 필요한 것: Spring, Spring MVC, Tomcat, JSON conversion...
-> Spring Boot Starter Web (웹 애플리케이션 & REST API 의존성 디스크립터) - 단위 테스트 작성에 필요한 것: Spring Test, JUnit, Mockito,...
-> Spring Boot Starter Test (단위 테스트 의존성 디스크립터) - 웹 애플리케이션 빌드 등, 다양한 기능에 필요한 의존성 descriptor(디스크립터)를 제공
- REST API 빌드할 때 필요한 것: Spring, Spring MVC, Tomcat, JSON conversion...
- Spring Boot Auto Configuration : 클래스 경로에 있는 의존성에 따라 자동으로 설정이 제공
- 애플리케이션을 빌드할 때 필요한 설정들: ComponentScan, DispatcherServlet, Data Sources, JSON Conversion, ...
- 애플리케이션을 빌드할 때 필요한 설정을 간소화: spring-boot-autoconfigure.jar에 정의된 설정을 토대로(혹은 오버라이드)
- application.properties 를 통해 간편한 설정 가능
- Spring Boot DevTools : 수동으로 서버를 다시 시작하지 않고도 애플리케이션을 변경 가능
- 코드를 수정하고, 애플리케이션을 자동으로 재시작하여 개발자의 생산성을 높일 수 있음
- pom.xml 수정 시 수동으로 재시작해야함
2. 프로덕션 환경에 빌드
- Logging
- 여러 환경에 맞는 다양한 설정 제공: Profiles, ConfiguraionProperties
- Monitoring (Spring Boot Actuator) : 메모리가 충분한지 애플리케이션의 측정항목을 살펴볼 수 있어야 함
이처럼 Spring Boot는 애플리케이션을 프로덕션 가능한 상태로, 매우 빠르게 빌드하는 데 도움이 되는 여러 기능을 제공합니다.
프로젝트 생성
먼저 Spring Boot 프로젝트를 생성합니다.
https://ride-dev.tistory.com/26
[SpringFramework] 스프링프로젝트 생성하기
0. 앞서 스프링 프레임워크에 대해 학습하기 위해 스프링 프로젝트를 생성합니다. 1. 프로젝트 생성하기 - spring.io 더보기 start.spring.io에 접속하여 스프링 프로젝트를 생성합니다 설정은 다음과
ride-dev.tistory.com
Spring Web 의존성을 추가합니다.
pom.xml에서 Spring Web의 의존성을 확인할 수 있습니다.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
IDE에서 프로젝트를 실행하여 정상적으로 작동하는지 확인합니다.
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v3.2.0)
2023-12-21T21:16:26.526+09:00 INFO 54576 --- [ main] c.s.s.l.LearnSpringBootApplication : Starting LearnSpringBootApplication using Java 17.0.6 with PID 54576 (/Users/sj/Desktop/projects/learn-spring-boot/target/classes started by sj in /Users/sj/Desktop/projects/learn-spring-boot)
2023-12-21T21:16:26.532+09:00 INFO 54576 --- [ main] c.s.s.l.LearnSpringBootApplication : No active profile set, falling back to 1 default profile: "default"
2023-12-21T21:16:27.795+09:00 INFO 54576 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port 8080 (http)
2023-12-21T21:16:27.805+09:00 INFO 54576 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2023-12-21T21:16:27.805+09:00 INFO 54576 --- [ main] o.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/10.1.16]
2023-12-21T21:16:27.859+09:00 INFO 54576 --- [ main] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
2023-12-21T21:16:27.861+09:00 INFO 54576 --- [ main] w.s.c.ServletWebServerApplicationContext : Root WebApplicationContext: initialization completed in 1246 ms
2023-12-21T21:16:28.307+09:00 INFO 54576 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port 8080 (http) with context path ''
2023-12-21T21:16:28.319+09:00 INFO 54576 --- [ main] c.s.s.l.LearnSpringBootApplication : Started LearnSpringBootApplication in 2.294 seconds (process running for 3.037)
Console에서
Spring Boot 및 Java 버전, 프로젝트 경로, port 등이 출력되는 것을 확인할 수 있습니다.
REST API 생성
이제 간단한 REST API를 생성해 보겠습니다.
http://localhost:8080/courses로 접속 시,
Course에서 id, name, author를 JSON으로 반환하도록 만들겠습니다.
[
{
"id": 1,
"name": "Learn Spring Boot",
"author": "ride-dev"
}
]
Course 객체를 만듭니다.
public class Course {
private long id;
private String name;
private String author;
public Course(long id, String name, String author) {
this.id = id;
this.name = name;
this.author = author;
}
public long getId() {
return id;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
@Override
public String toString() {
return "Course{" +
"id=" + id +
", name='" + name + '\'' +
", author='" + author + '\'' +
'}';
}
}
Controller를 생성하고 URL을 매핑합니다.
@RestController
public class CourseController {
@RequestMapping("/courses") // URL을 특정 메소드에 매핑
public List<Course> retrieveAllCourses() {
return Arrays.asList(
new Course(1, "Learn Spring", "ride-dev"),
new Course(2, "Learn Spring Boot", "ride-dev")
);
}
}
localhost:8080/courses에 접속하여 확인합니다.
JSON 포맷팅
크롬 확장프로그램인 JSON Formatter을 사용하면 JSON을 더 직관적으로 확인할 수 있습니다.
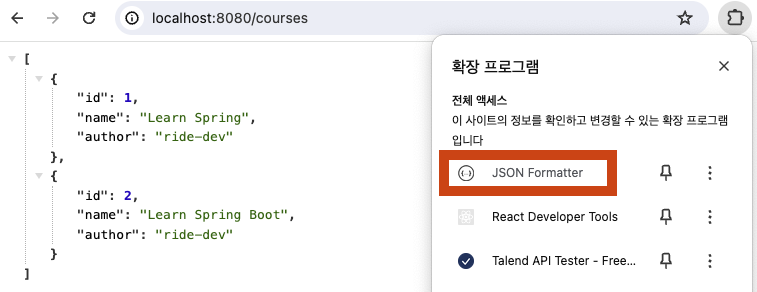
이렇게 Spring Boot 프로젝트를 생성하고, 간단한 REST API를 구현해 볼 수 있었습니다.
'Java' 카테고리의 다른 글
[SpringBoot] Spring Boot vs. Spring vs. Spring MVC (0) | 2023.12.22 |
---|---|
[SpringBoot] 프로덕션 환경 배포 준비하기(로깅, 모니터링, 구성관리) (0) | 2023.12.22 |
[SpringBoot] Spring Boot 등장 배경 (1) | 2023.12.21 |
[SpringFramework] 개념 및 용어 정리 (0) | 2023.12.20 |
[SpringFramework] Spring Annotations (1) | 2023.12.20 |